How to properly document code?

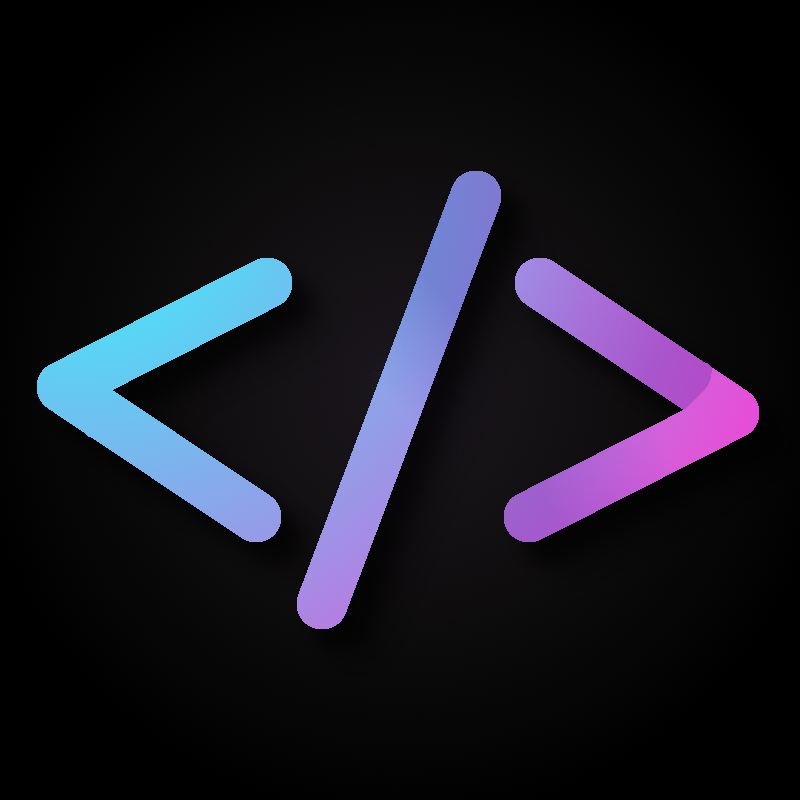
I just recently started documenting my code as it helped me. Though I feel like my documentations are a bit too verbose and probably unneeded on obvious parts of my code.
So I started commenting above a few lines of code and explain it in a short sentence what I do or why I do that, then leave a space under it for the next line so it is easier to read.
What do you think about this?
Edit: real code example from one of my projects:
async def discord_login_callback(request: HttpRequest) -> HttpResponseRedirect:
async def exchange_oauth2_code(code: str) -> str | None:
data = {
'grant_type': 'authorization_code',
'code': code,
'redirect_uri': OAUTH2_REDIRECT_URI
}
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
async with httpx.AsyncClient() as client:
# get user's access and refresh tokens
response = await client.post(f"{BASE_API_URI}/oauth2/token", data=data, headers=headers, auth=(CLIENT_ID, CLIENT_SECRET))
if response.status_code == 200:
access_token, refresh_token = response.json()["access_token"], response.json()["refresh_token"]
# get user data via discord's api
user_data = await client.get(f"{BASE_API_URI}/users/@me", headers={"Authorization": f"Bearer {access_token}"})
user_data = user_data.json()
user_data.update({"access_token": access_token, "refresh_token": refresh_token}) # add tokens to user_data
return user_data, None
else:
# if any error occurs, return error context
context = generate_error_dictionary("An error occurred while trying to get user's access and refresh tokens", f"Response Status: {response.status_code}\nError: {response.content}")
return None, context
code = request.GET.get("code")
user, context = await exchange_oauth2_code(code)
# login if user's discord user data is returned
if user:
discord_user = await aauthenticate(request, user=user)
await alogin(request, user=discord_user, backend="index.auth.DiscordAuthenticationBackend")
return redirect("index")
else:
return render(request, "index/errorPage.html", context)
You are viewing a single comment
Your code should generally be self documenting: Have variable and method names that make sense.
Use comments when you need to explain something that might not be obvious to someone reading the code.
Also have documentation for your APIs: The interfaces between your components.
One interesting thing I read was that commenting code can be considered a code smell. It doesn't mean it's bad, it just means if you find yourself having to do it you should ask yourself if there's a better way to write the code so the comment isn't needed. Mostly you can but sometimes you can't.
API docs are also an exception imo especially if they are used to generate public facing documentation for someone who may not want to read your code.
Agree with you though, generally people should be able to understand what's going on by reading your code and tests.
Great points. I'm a huge advocate for adding comments liberally, and then treating them as a code smell after.
During my team's code reviews, anything that gets a comment invariably raises a "could we improve this so the comment isn't need?" conversation.
Our solution is often an added test, because the comment was there to warn future developers not to make the same mistake we did.
I know there are documentation generators (like JSDoc in JavaScript) where you can literally write documentation in your code and have a documentation site auto-generated at each deployment. There’s definitely mixed views on this though
To my knowledge that just formats existing comments. With LLMs you could probably do 95% of the actual commenting.
Useful comments should provide context or information not already available in the code. There is no LLM that can generate good comments from the source alone
Codium does surprisingly well at generating JSDoc, and it processes your code within the context of your entire codebase. Still not quite there yet, but you might be surprised
Why wouldn’t it be able to? It can link similar code structure to data in its training set. Maybe the ones that aren’t at that level but it’s hardly a stretch to make these inferences. Most of the code you write is hardly novel.
If it’s not exactly novel, how many comments do you really need?
An LLM is just gonna describe the code it sees. Good comments should include information and context that is not already in the source.
I’m mostly talking about when you need to use JSDoc format which are usually for interfaces, so it’s usually just a chore for humans.
Probably harder to get good comments inside code, but it might still be possible.