How do you directly interface with GPIO in Python?
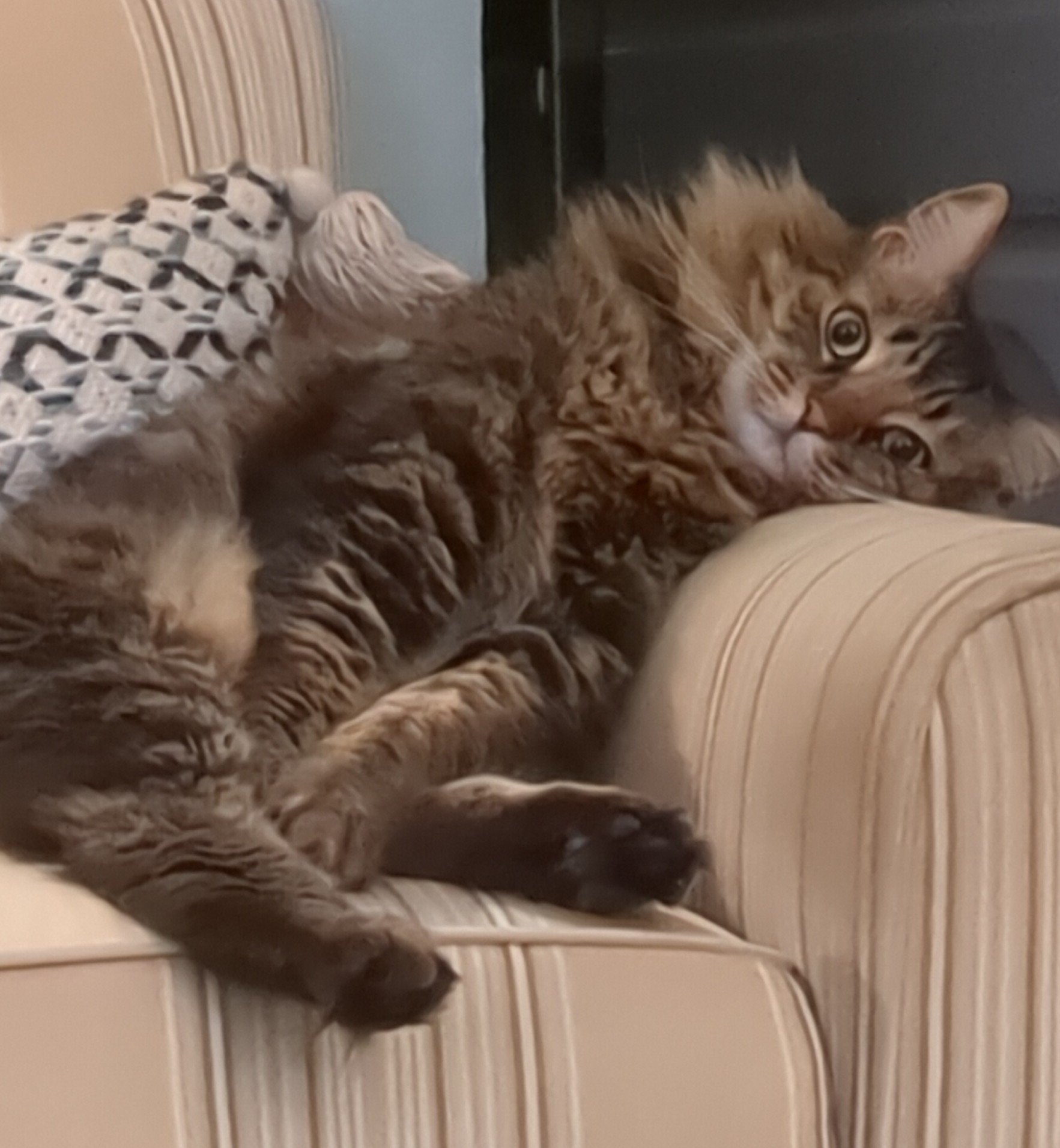
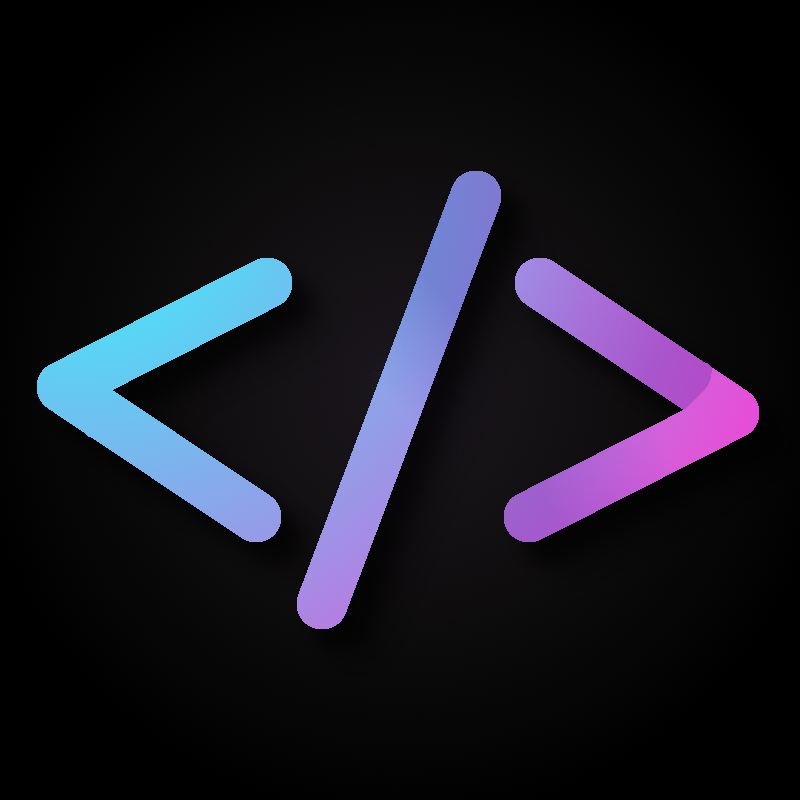
Like let's say I have a few old HP alphanumeric LED displays that have a simple bit pattern protocol. I've gotten them working in Arduino a long time ago. If I can find some unused pins how can I bit bang them into a custom protocol from user space using pins that may be unrelated as far as I/O ports on a modern computer? Is it even possible without a kernel module? Am I stuck with using a serial channel like SPI/I2C/UART to talk to an external controller?
You don't need any garbage 3rd party packages. Just use ctypes.
Read the documentation for ctypes. You can use any native object in Python the way you do in C using ctypes.
Whatever you do, don't trust some god-forsaken 3rd party package. I think people have forgotten to be clever and resourceful these days. A binary object is itself a package, why would you use s another package to communicate with it?
Here's how you can import libc into Python:
Just pass it path to the binary object that holds the symbols for GPIO.
Some tricks. Here's how you can use
libc
to make a syscall in Python:You can use WiringPI to control the GPIOs from Python
Could you use a small microcontroller running micropython? Then you can just type into the repl on that over usb, or run your python on it directly
I've done that before, and with Forth. I'm more interested in bridging what I know with hardware into the desktop environment... or understanding why it seems so disconnected.
Possibly important detail - what type of computer do you propose running this? Most methods that are common if you search the internet or ask here will likely apply to Raspberry Pi and it's clones, but if you have something more esoteric it might not work.